Python is a key language in DevOps due to its versatility, ease of learning, and powerful libraries. It allows DevOps engineers to automate workflows, manage infrastructure, and deploy applications efficiently. Here’s an outline of how Python is used in DevOps
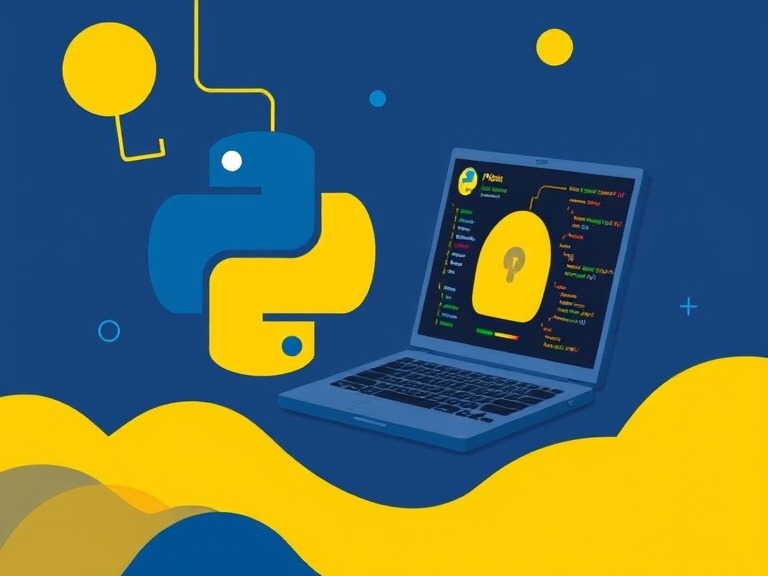
Key Use Cases of Python in DevOps
1. Automation
- Writing scripts to automate repetitive tasks like log file parsing, backups, or monitoring.
- Example: Automating server restarts or application deployment.
import os
os.system("sudo systemctl restart nginx")
2. Infrastructure as Code (IaC)
- Tools like Ansible and Terraform can be extended using Python.
- Directly managing infrastructure resources via libraries like boto3 (AWS SDK for Python).
import boto3
ec2 = boto3.resource('ec2')
instance = ec2.create_instances(
ImageId='ami-0abcd1234abcd5678',
MinCount=1,
MaxCount=1,
InstanceType='t2.micro'
)
print(f'Launched instance: {instance[0].id}')
3. Configuration Management
- Automating system configurations and ensuring consistent environments using Python.
- Example: Writing scripts to configure servers during provisioning.
import subprocess
commands = [
"sudo apt update",
"sudo apt install -y nginx",
"sudo ufw allow 'Nginx HTTP'"
]
for command in commands:
subprocess.run(command, shell=True)
4. Continuous Integration and Continuous Deployment (CI/CD)
- Integrating Python scripts into CI/CD pipelines (e.g., Jenkins, GitLab CI).
- Writing Python scripts for build, test, or deployment automation.
import os
# Trigger a deployment
os.system("git pull origin main")
os.system("docker-compose up --build -d")
5. Containerization and Orchestration
- Managing Docker containers and Kubernetes clusters using Python libraries.
- Example: Using
docker
orkubernetes
Python SDKs.
from docker import from_env
client = from_env()
container = client.containers.run("nginx", detach=True, ports={"80/tcp": 8080})
print(f'Container {container.id} started on port 8080.')
6. Monitoring and Logging
- Creating custom monitoring tools using libraries like psutil for system resource usage.
- Parsing logs with regex or libraries like loguru.
import psutil
cpu_usage = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
print(f"CPU Usage: {cpu_usage}%")
print(f"Available Memory: {memory_info.available // (1024 * 1024)} MB")
7. Cloud Management
- Managing cloud services (AWS, Azure, GCP) with Python SDKs.
- Automating tasks like creating VM instances, managing storage, and deploying resources.
from google.cloud import storage
client = storage.Client()
bucket = client.create_bucket('my-devops-bucket')
print(f'Bucket {bucket.name} created.')
8. Testing and Validation
- Automating testing of applications and environments.
- Tools like pytest, unittest, and selenium for functional and performance testing.
import pytest
def test_sum():
assert sum([1, 2, 3]) == 6
Popular Python Libraries for DevOps
1. Infrastructure and Cloud Management:
- boto3: AWS automation.
- google-cloud: GCP management.
- azure-mgmt-resource: Azure management.
2. Automation:
- Fabric: Remote server automation.
- Paramiko: SSH and SFTP.
3. CI/CD:
- JenkinsAPI: Jenkins integration.
- GitPython: Automating Git operations.
4. Monitoring:
- psutil: System monitoring.
- Prometheus-client: Custom Prometheus metrics.
Python in a DevOps Workflow
1. Infrastructure Setup
- Provision resources with Python scripts or SDKs.
- Example: Automate setting up EC2 instances, S3 buckets, and RDS databases.
2. Configuration Management
- Ensure uniform environments using Python automation tools.
3. Deployment
- Automate deployment pipelines with Python in CI/CD systems.
4. Post-Deployment Monitoring
- Monitor application performance and alert on issues using Python scripts.
You May Also Like
How to Set Up a Virtual Private Cloud (VPC) on AWS: A Step-by-Step Guide